Getting started
You may be familiar with quick-sort, but when you stumble across this problem, it turns out to be a lot more complex. Since you need to determine the method that involves the fewest moves possible, you will have to take another approach. The given problem can be re-stated into this problem:
Problem statement
Hien is the class monitor and he wants his classmates to form a line, in which the height of every students is in ascending order. He needs to form that line by moving his classmates from the line to the start or end of it and it has to be a quick process, since Hien is very lazy and needs to play Age Of Empires right away. Write an algorithm to help him.
Input format
First line: n.
Second line: n numbers indicating the height of every student in the class, each seperated by a space.
Constraints
n ≤ 100; H[i] ≤ 100000 (H[i] is the height of an individual).
Output format
An integer indicating the fewest moves possible.
Sample input
4
2 1 3 5
Sample output
1
Explanation
The student with height 1 is moved to the start of the line.
Let’s not pay attention to the ‘fewest moves’ for a while. Normally, when you see these types of ‘moving’ elements to start or end of an array, you can take a look at a basic approach.
Let’s take the Sample input as an example. With the basic approach, we search for the smallest element in the array, which is now 1. After that, we move it to the far right of the array. Then, we search for the next smallest element, which is 2, and we keep doing it until 5 is moved to the far right of the array. We come to the conclusion that for this approach, the number of moves that are taken is exactly equal to the number of elements present in the array itself. But let’s have a closer look. We can see that 2, 3 and 5 are contiguous, meaning that the relative order between them is not changed at all when sorting is completed. So, we can know that in the required algorithm, we need to conserve the order of contiguous integers. That is when std::pair comes to use.
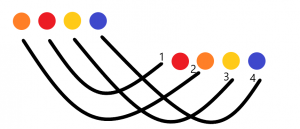
What is std::pair, exactly?
Std::pair is a pre-defined class in C++. A pair element is consisted of 2 other sub-elements, which can be classified as first and second. In the algorithm we are searching for, as stated earlier, we can utilise std::pair to get the job done by assigning the input elements to first and its index to second.
Get the job done
After that, quick-sort comes in handy. We then use it to sort the array in ascending order. Because std::pair is used, when sorting the elements, each index is carried along with the data. Then, we can just compare the indexes of every subsequent element. Job done!
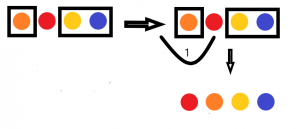
Source code (C++)